Viewing Job Runs
TrueFoundry provides two methods for viewing job runs: through the user interface (UI) or programmatically using either the Python SDK
or the servicefoundry CLI
.
Viewing Job Runs via User Interface
Viewing Job Runs Programmatically
TrueFoundry's Python SDK and servicefoundry (CLI) provide programmatic ways to retrieve job runs. You can use these to list all job runs or view the details of a specific job run.
Prerequisites
To view job runs programmatically, you will need the following information:
-
Application FQN: The Fully Qualified Name (FQN) of the job you want to view job runs for.
-
Job Run Name: The name of the specific job run you want to view.
You can obtain this information from the Job-specific dashboard of the Job you are interested in.
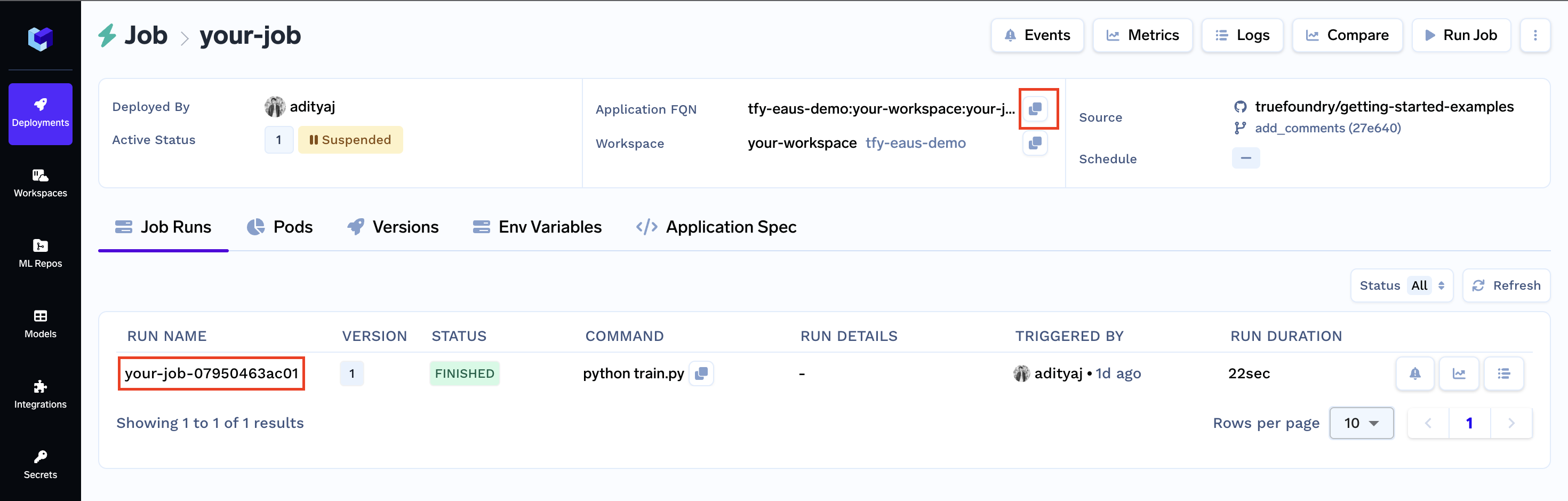
Viewing List of Job Runs Programmatically
Given a Job, there are two ways of seeing its list of job runs programmatically: using the servicefoundry
CLI or the TrueFoundry Python SDK
.
Command-Line Interface (CLI)
servicefoundry list job-run --application-fqn <your-application-fqn>
Python SDK
from servicefoundry import list_job_runs
application_fqn = "<your-application-fqn>"
job_runs = list_job_runs(application_fqn=application_fqn)
print("Number of Job Runs:", len(job_runs))
for run in job_runs:
print(f"Job name: {run.name}, status: {run.status}, command: {run.command}\n")
Viewing a Specific Job Run Programmatically
Just like viewing a list of job runs, there are two ways of extracting a specific job run programmatically: using the servicefoundry
CLI or the TrueFoundry Python SDK
. Apart from the Application FQN
, the Job Run Name
will be used to extract a unique job run.
Command-Line Interface (CLI)
servicefoundry get job-run --application-fqn <your-application-fqn> --job-run-name <your-job-run-name>
Python SDK
from servicefoundry import get_job_run
application_fqn = "<your-application-fqn>"
job_run_name = "<your-job-run-name>"
job_run = get_job_run(application_fqn=application_fqn, job_run_name=job_run_name)
print(f"Job name: {job_run.name}, status: {job_run.status}, command: {job_run.command}")
Updated 5 months ago